March 11th, 2019 | by Tomasz Jarzyński
How to Store Sensitive Data of Your Application in .NET Core App?
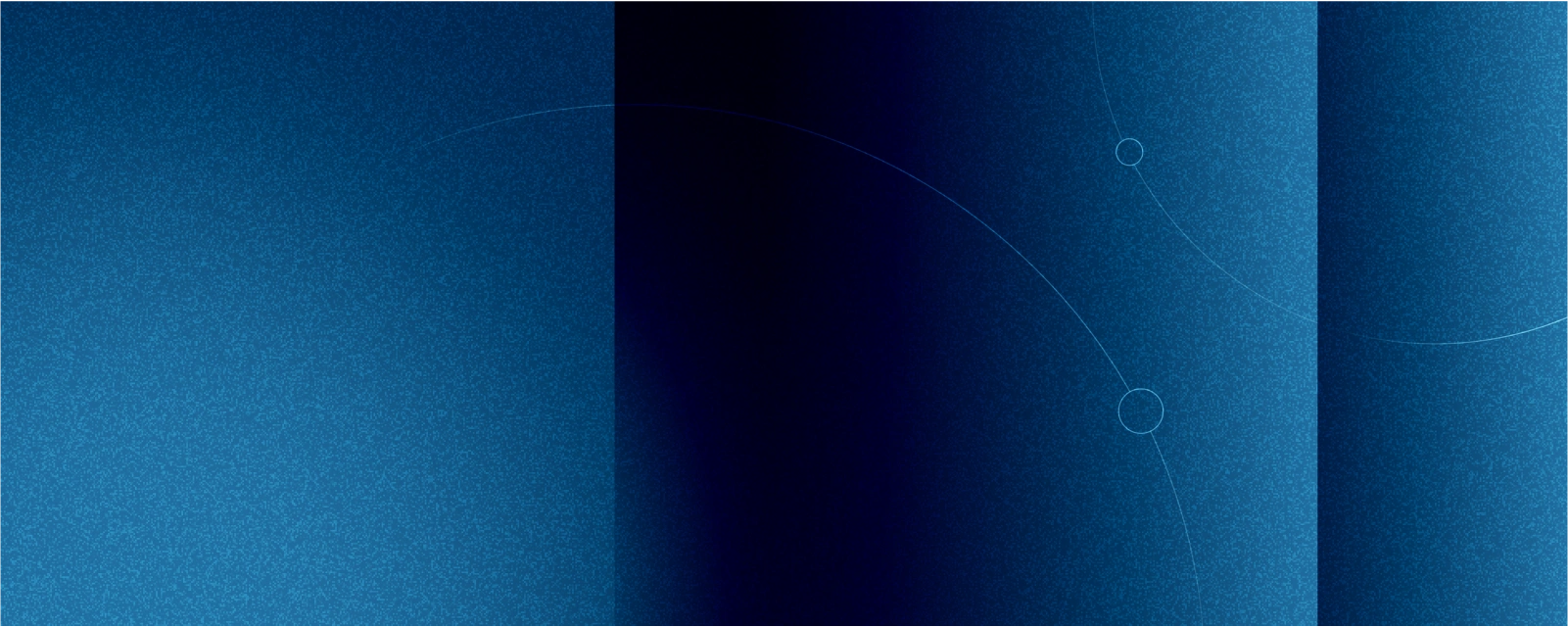
Table of contents
Imagine that you are working on your pet project on your localhost. After one month you have created a small diamond. You want to publish it on the internet and still maintain your solution. Do you want to share your code on GitHub to show everyone what you have? Created and get thousands of stars on GitHub. Sounds nice, doesn’t it? But let us stay on the ground and focus on the first problem which you can meet on the long way to your glory.
Imagine that you created your fantabulous app on localhost. Your app needs to connect to a database. How can you store your connection string?
- The ugliest way will be to just hardcode that:
public void ConfigureServices(IServiceCollection services) { services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1); var connectionString = “Server=myServer; Port=3306; Database=myDatabase; Uid=myUser; Pwd=myPassword;”; services.AddDbContext<MyAppContext>(o => o.UseMySql(connectionString)); }
Unfortunately, it wouldn’t be the best idea to commit code like that to GitHub. Everyone could see all your sensitive data.
Microsoft, in that case, suggests using Secret Manager. Secret Manager creates a user secret file. How can we create the file?
- Right-click on the project ➡ Manage User Secrets.

- You will be navigated to a new file secret.json. The file hasn’t been added to the solution. Why? To prevent the user checking in that file to source control. Another question is, where is the file? It depends on your OS:
Windows: %APPDATA%MicrosoftUserSecrets<user_secrets_id>secrets.json
macOS: ~/.microsoft/usersecrets/<user_secrets_id>/secrets.json
Linux: ~/.microsoft/usersecrets/<user_secrets_id>/secrets.json
- To find out your <user_secrets_id>, you need to edit csproj:

The code of usersecret.csproj:
<Project Sdk=”Microsoft.NET.Sdk.Web”> <PropertyGroup> <TargetFramework>netcoreapp2.1</TargetFramework> <UserSecretsId>8a80a183-58cb-4491-99fc-4adaeb9e2228</UserSecretsId> </PropertyGroup> <ItemGroup> <Folder Include=”wwwroot” /> </ItemGroup> <ItemGroup> <PackageReference Include=”Microsoft.AspNetCore.App” /> <PackageReference Include=”Microsoft.AspNetCore.Razor.Design” Version=”2.1.2″ PrivateAssets=”All” /> </ItemGroup> </Project>
As you can see we have the node UserSecretsId. So if you use Windows, your secret.json will be under the location:
MicrosoftUserSecrets8a80a183-58cb-4491-99fc-4adaeb9e2228secrets.json
- Now you can edit your secret.json:
{ “dev”: { “connectionString”: “Server=myServer; Port=3306; Database=myDatabase; Uid=myUser; Pwd=myPassword;” } }
- Now you have to inform the compiler that it can use the user secret file.
public void Configure(IApplicationBuilder app, IHostingEnvironment env) { var builder = new ConfigurationBuilder().AddUserSecrets<Startup>(); if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } app.UseMvc(); }
- To access your secret key just write the code:
public void ConfigureServices(IServiceCollection services) { services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1); var connectionString = Configuration[“dev:connectionString”]; services.AddDbContext<SongContext>(o => o.UseMySql(connectionString)); }
Right now you can commit your code without any hesitation if somebody could steal your sensitive data.
Read the next article: OAuth 2.0 – What Is An Authorization?